The Art of Code Design: Embracing the Power of the Open-Closed Principle
Coding (Software architecture)
Discover the power of the Open-Closed Principle and learn how to build flexible and adaptable software.
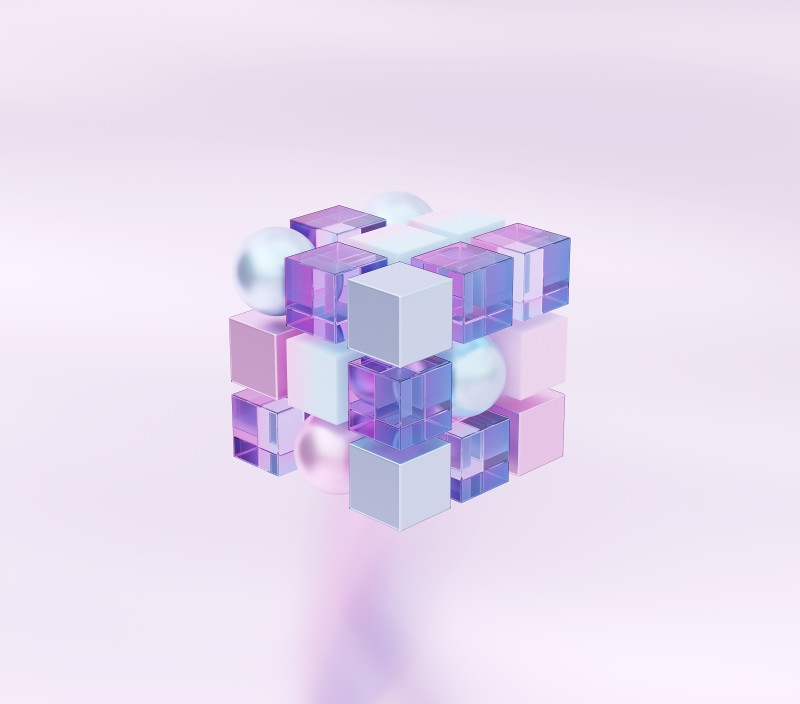
As web developers, we know the importance of writing clean and maintainable code.
That's where the Open-Closed Principle (OCP) comes in.
It's all about creating code that is open for extension but closed for modification.
By following this principle, we can build software that is adaptable, scalable, and easier to maintain.
In this blog post, we'll explore the essence of the Open-Closed Principle, uncover its benefits, and learn how to apply it in real-world scenarios.
Let's dive into the world of the Open-Closed Principle and unlock the art of code design. Happy coding and let's get started!
Understanding the Open-Closed Principle
The Open-Closed Principle (OCP) is a fundamental principle in object-oriented programming that guides us in designing software components that are open for extension but closed for modification.
At its core, the principle promotes the idea of building systems that are flexible and easily adaptable to change without the need to modify existing code.
The essence of the Open-Closed Principle lies in separating the behavior of a module or class from its implementation details.
In other words, when a new requirement or feature emerges, we should strive to extend the functionality of our code rather than modify the existing codebase.
This approach allows us to minimize the risk of introducing bugs or unintended side effects in the existing code while adding new functionality.
By adhering to the Open-Closed Principle, we aim to create code that is resilient to change and promotes a modular and cohesive architecture.
It encourages the use of abstraction, encapsulation, and polymorphism to ensure that modules or classes are easily extendable by adding new behaviors or variations without altering their existing implementation.
The core idea behind the Open-Closed Principle is to design our code in a way that new functionalities can be added by writing new code rather than modifying existing code.
This also enhances the maintainability and reusability of our codebase.
By embracing the Open-Closed Principle, we can achieve a higher degree of flexibility, scalability, and maintainability in our software projects.
The OCP in Practice
The Open-Closed Principle (OCP) promotes code extensibility and, as I wrote above, maintainability by emphasizing "open for extension, closed for modification."
It encourages adding new functionality without directly modifying existing code.
By using abstraction and interfaces, we create modules that can be extended.
The OCP fosters code reusability and modularity, allowing independent development and seamless integration of extensions.
Applying the OCP with Abstraction and Polymorphism
When applying the Open-Closed Principle (OCP), abstraction and polymorphism play a vital role.
Abstraction involves creating abstract classes or interfaces that define the common behavior or contract for a group of related classes.
Polymorphism, on the other hand, allows objects of different concrete classes to be treated interchangeably through their shared interfaces.
To adhere to the OCP, we can create an abstract class or interface that represents the common behavior of a set of related classes.
I understand what I am writing can be pretty confusing so let's take an example of a shape hierarchy: Circle, Square, and Triangle.
We can define an abstract Shape class or an interface, such as `ShapeInterface`, that includes common methods like `calculateArea` and `render`.
abstract class Shape { abstract public function calculateArea(): float; abstract public function render(): void; } class Circle extends Shape { // implementation of calculateArea and render specific to Circle } class Square extends Shape { // implementation of calculateArea and render specific to Square } class Triangle extends Shape { // implementation of calculateArea and render specific to Triangle }
Now, by adhering to the OCP, we can introduce new shapes without modifying existing code.
For instance, if we want to add a Rectangle shape, we can simply create a new class that extends the abstract Shape class and implements the required methods.
class Rectangle extends Shape { // implementation of calculateArea and render specific to Rectangle }
With abstraction and polymorphism, we can write code that relies on the common `Shape` abstraction and works seamlessly with any concrete shape class.
This allows us to introduce new shapes without modifying existing code, keeping our system open for extension.
By leveraging the power of interfaces and abstract classes, we achieve flexible code, and maintainability, and adhere to the Open-Closed Principle.
Do you see?
A piece of cake!
Let's see how to use this in detail now.
Using Interfaces and Abstract Classes
As you have just seen interfaces and abstract classes play a crucial role in implementing the Open-Closed Principle (OCP) and promoting code flexibility and modularity.
Interfaces define a contract that a class must adhere to by specifying the required methods.
They allow different classes to implement the same interface, enabling polymorphism and interchangeability.
In our example of shapes, we can create an interface called `ShapeInterface` that declares the common methods like `calculateArea` and `render`.
interface ShapeInterface { public function calculateArea(): float; public function render(): void; }
By implementing the `ShapeInterface`, concrete shape classes like Circle, Square, Triangle, and Rectangle guarantee that they provide the necessary functionality defined in the interface.
This allows us to treat instances of these classes interchangeably when working with code that relies on the `ShapeInterface`.
Abstract classes, on the other hand, provide a way to define common behavior and partially implement methods.
They can serve as a base for other classes to inherit from while also allowing extension and customization.
In our example, we can have an abstract class called `AbstractShape` that implements the `ShapeInterface` and provides a default implementation for the `render` method.
abstract class AbstractShape implements ShapeInterface { public function render(): void { // Default rendering implementation } }
Concrete shape classes can then extend the `AbstractShape` class, inheriting the default implementation of the `render` method while still having the flexibility to provide their specific implementation of the `calculateArea` method.
Using interfaces and abstract classes in the context of the OCP allows us to define contracts, enforce behavior, and provide a framework for extension.
By coding to interfaces and utilizing abstract classes, we create modular and flexible systems that can easily accommodate new shapes or modifications without the need to modify existing code.
Applying Design Patterns that Align with the OCP
Design patterns provide proven solutions to common software design problems, and several of them align perfectly with the Open-Closed Principle (OCP).
Two such design patterns are the Strategy pattern and the Decorator pattern.
The Strategy pattern (I have written about it already) allows us to encapsulate interchangeable behaviors behind a common interface.
In the context of our shape example, we can define a `CalculationStrategyInterface` that declares a method `calculateArea` and have different strategies, such as `CircleCalculationStrategy`, `SquareCalculationStrategy`, etc., that implement this interface.
interface CalculationStrategyInterface { public function calculateArea(ShapeInterface $shape): float; } class CircleCalculationStrategy implements CalculationStrategyInterface { public function calculateArea(ShapeInterface $shape): float { // Calculate the area for a circle } } // Similarly, define strategies for other shapes
By utilizing the Strategy pattern, we can dynamically assign different calculation strategies to shape objects without modifying the existing code.
This allows us to add new calculation strategies or modify existing ones without impacting the core shape classes.
The Decorator pattern provides a way to add new functionality to an object dynamically.
In our shape example, we can have a `ShapeDecorator` abstract class that extends `AbstractShape` and implements the `ShapeInterface`.
This class acts as a base for concrete decorators like `ColorDecorator` or `BorderDecorator`, which add additional features to the shapes.
abstract class ShapeDecorator extends AbstractShape { protected $shape; public function __construct(ShapeInterface $shape) { $this->shape = $shape; } } class ColorDecorator extends ShapeDecorator { public function render(): void { // Additional rendering code for color $this->shape->render(); } } // Similarly, define decorators for other additional features
By applying the Decorator pattern, we can wrap our shape objects with different decorators to add new functionalities dynamically.
This allows us to extend the behavior of shapes without modifying their core implementation, ensuring compliance with the OCP.
Both the Strategy pattern and the Decorator pattern adhere to the Open-Closed Principle by enabling the addition of new functionality without modifying existing code.
They provide modular and flexible solutions that align with the principles of object-oriented design and allow for easier maintenance and extension of software systems.
Challenges and Considerations
Implementing the Open-Closed Principle (OCP) comes with certain challenges and considerations.
Finding the right level of abstraction and identifying proper extension points can be challenging.
The initial design effort and ongoing maintenance require discipline and adherence to best practices.
Strict adherence to the OCP can introduce complexity and potential performance impacts.
However, by overcoming these challenges, developers can achieve more flexible and maintainable codebases that allow for easier modifications and additions.
I have found that it all depends on how comfortable are you using these principles and design patterns.
The more you practice the easier it will get.
If you have any questions subscribe to my newsletter and contact me afterward, I'll be more than happy to answer or create an article for you.
Conclusion
We have explored the power of the Open-Closed Principle (OCP) in software development.
We discussed its core idea of being "open for extension, closed for modification" and how it promotes code extensibility and maintainability.
By leveraging abstractions, polymorphism, and design patterns aligned with the OCP, we can create flexible and modular code that is easier to modify and extend.
I encourage you, to apply the OCP to your projects.
Embrace the mindset of designing code that is open to change without the need for extensive modifications.
By adhering to the OCP, you can future-proof your applications, save time and effort in maintenance, and foster better collaboration within development teams.
Stay tuned for upcoming blog posts in our SOLID principles series, where we will dive into other important principles that contribute to high-quality software design.
And don't forget to subscribe to our newsletter to receive free reviews of the best books on web development.
Expand your knowledge and stay up-to-date with the latest insights and techniques in the world of programming.
Thank you for joining me on this journey towards mastering the art of code design.
Together, let's create robust and elegant solutions that stand the test of time.
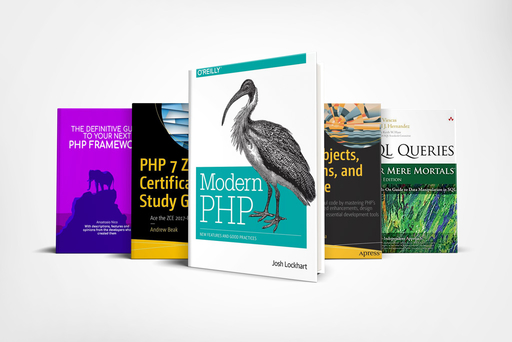