PHP 7.4 (Changes & Performance)
Coding (Php 7.x)
Last chapter of the series about PHP 7.4, in this one you will see changes in PDO, Composer, Benchmark plus JIT and more
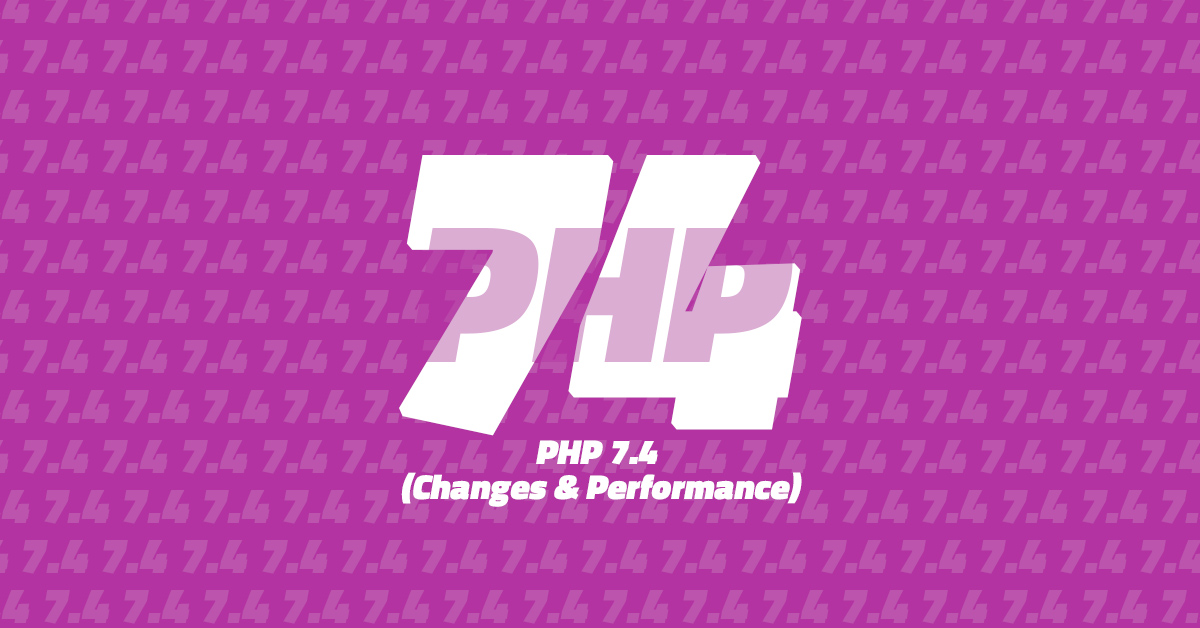
Introduction
PHP has been released the 28th October 2019 and it is the last 7.*’s version of this programming language.
The idea behind PHP 7.4 is to get the developers ready for PHP 8.
So, given that this version is just a preview of PHP 8 the question is:
Why don’t just wait for it rather than upgrade now?
PHP 7.4 offers the possibility to get the habit of using more ‘forward-looking’ features.
With the implementation of typed variables, preloading, a better syntax for array functions as so on.
It also provides better performance than the older versions.
And most important, the release date of PHP 8 is on September 2021.
After reading this post you are going to have a complete and more clear idea about PHP 7.4, its speed and the new toys that are included in it.
Then we are going to learn the rumours and what has leaked about PHP 8 so far.
The series
This post is part of the series;
We have already seen all the new features of PHP 7.4 and how to get your code ready by discovering all the deprecations
Other changes
Let’s start with the changes that did not make it in the previous episodes.
PEAR is not enabled by default anymore
What is PEAR?
It is a package manager for PHP, The aim of PEAR is (maybe better writing was?) to provide reusable components to PHP developers.
It was the precursor of what we now call Composer, the PEAR project is not just old it is ancient, you can see tutorial 10 years old still making on the first page of Google.
This means that the majority of the code on it is outdated.
Unlike Composer, every package in PEAR needed to be reviewed before being published, which was a good thing, but it limited the number of packages available.
Another problem was that every package was installed globally rather than depending on the project.
In this post, Fabien Potencier, the author of Symphony, described the life of PEAR and its downfall over Composer.
What is happening now?
One of the goals of PHP 7.4 was to be less cluttered, a way to do so was to remove superfluous code from the core engine.
As you saw in the previous paragraph PEAR didn't had so much future, especially compared with its more modern alternative, Composer.
Sooner or later this project would have been deprecated.
For the first time now, when you install PHP 7.4, your environment is not going to have PEAR enabled automatically.
If you need it you can still enable it using the command --with-pear but this is a deprecated option that will likely be removed in PHP 8 already.
If you can choose, prefer Composer
PDO
Consider PDO as an approach you can take to access databases on your web applications.
PDO is a layer that is in front of an actual database language to make your queries and databases’ command more consistent.
PDO supports a lot of different databases to be connected with PHP:
- Cubrid
- FreeTDS / Microsoft SQL Server / Sybase
- Firebird
- IBM DB2
- IBM Informix Dynamic Server
- MySQL 3.x/4.x/5.x
- Oracle Call Interface
- ODBC v3 (IBM DB2, unixODBC and win32 ODBC)
- PostgreSQL
- SQLite 3 and SQLite 2
- Microsoft SQL Server / SQL Azure
- 4D
Here is what I believe is the best article about PDO
In this last version of PHP, there have been several small changes occurred in regards to PDO,
User and password added to driver invocation
Until 7.3 to connect to a database you would have being done like this:
/* Connect to a MySQL database using driver invocation */ $dsn = 'mysql:dbname=database_name;host=127.0.0.1'; $user = 'database_user'; $password = 'database_pass'; try { $dbh = new PDO($dsn, $user, $password); } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); }
In here you are invoking a driver then check the connection using the credential of the user.
If you connect to MySQL, mssql, Sybase, dblib, firebird and oci drivers you can now avoid the user assignment lines and all in the din variable.
If the credentials are specified both in the constructor and the $dns, the constructor takes precedence.
/* Connect to a MySQL database using driver invocation */ $dsn = 'mysql:host=127.0.0.1;port=3306;dbname=database_name;user=database_user;password=database_pass'; try { $dbh = new PDO($dsn); } catch (PDOException $e) { echo 'Connection failed: ' . $e->getMessage(); }
Escape PDO "?" parameter placeholder
PostgreSQL allows the usage of the keyword "?" (question mark) character in operations.
Until PHP 7.3 it was impossible to use this keyword with the PHP extension.
The reason was that "?" is used a placeholder and could not be escaped.
Matteo Beccati’s proposal wat to enhance the SQL parser of PDO and allow question marks to be escaped by doubling them.
In this way the “??” string is understood and interpreted as “?”, instead of the single “?” is going to be interpreted as a positional parameter placeholder like previous versions.
$stmt = $pdo->prepare('SELECT * FROM users WHERE name ?? ?'); $stmt->execute(['Tommy']); SELECT * FROM users WHERE name ? 'Tommy'
PDO throwing Exception
Internal classes in PHP do not support serialization.
Since PDOException and PDO classes are internal classes they will now generate an Exception rather than a PDOException,
PDO and Oracle databases
PDO_OCI is the PDO driver for Oracle databases and its class PDOStatement has now a new method:
It is getColumnMeta().
This method returns an array with metadata for a column in a result set.
The keys of this array are native_type, flags, name, len, precision and pdo_type.
errorInfo() to show SQLite3 extended result
The function errorInfo() of the PDO class fetch errors’ information associated with the last operation executed on a database.
You can now enable the use of SQLite3 extended result codes after setting the attribute of the function setAttribute() as true.
PDO::setAttribute(PDO::SQLITE_ATTR_EXTENDED_RESULT_CODES, true)
PDO and SQLite in PHP 7.4
Two more updates regarding SQLite in this new version of PHP:
The flag --with-pdo-sqlite of PDO_SQLite used to accept a directory in which the SQLite base was installed now it does not accept a directory anymore
The method getAttribute() of the class PDOStatement retrieves the attributes of a database connection.
When the parameter is PDO::SQLITE_ATTR_READONLY_STATEMENT it now allows checking if the statement is read-only.
SQLite3
As we said in the previous paragraph we can now check whether the statement is read-only using PDOStatement::getAttribute(PDO::SQLITE_ATTR_READONLY_STATEMENT)
The other new feature is the SQLITE_ATTR_EXTENDED_RESULT_CODES on the setAttribute function.
We now have two more functions to our SQLite3 class:
getSQL() that retrieves the SQL of the statement and SQLite3::backup() that is used to create a backup database using the online backup API.
About SQLite3 class we also have the function lastExtendedErrorCode() that get the last extended result code,
enableExtendedResultCodes() that as we saw previously make the function lastErrorCode() return extended result codes
Other little changes are about the bundle libsqlite that has been removed and the unserialization of SQLite3.
SQLite3Stmt and SQLite3Result have been forbidden.
New Global Constants
We now have 36 new global constants that we can categorize in 4 different types:
- PHP Core
- Multibyte string, that supplies multibyte string functions to support you while dealing with multibyte encodings)
- Sockets, that is a service in PHP that includes a low-level interface to the socket communication functions, it relies on BSD sockets, giving the possibility to act as a socket server as well as a client
- Tidy, that is a basic extension for the Tidy HTML, it allows to clean HTML, XHTML, and XML and traverse the document tree
Changing on the RFC voting process
Two rules have changed when voting for an RFC proposal
- Now ⅔ is required for a proposal to pass
- All RFCs must be open for at least 2 weeks
Why PHP 7.4?
Legacy PHP is still around
Let’s stop with the technical stuff and let’s talk about language usage.
With data updated on the 29 of November, the website W3Techs.com shows that 78.9% of the website in the world run in PHP and 55.9% of these websites are still in PHP 5.
Just to put the thing in context PHP 7.0 has been released in December 2015 and was supported until the same month of 2018.
This is hugely concerning about security and data protection.
"PHP is dead" - anyone about PHP
If you browse the web you will see a lot’s of developers writing that PHP is dead and is not worth to learn it anymore,
well, the numbers say the opposite.
PHP applications are just not updated by the developers themselves.
That’s another reason why I am writing this series.
The benchmarks
What about the performances?
Michael Larabel, founder and principal author of Phoronix has tested various version of PHP this year also adding the development PHP 8.0 to the comparison,
And the result, even though the changes between the versions are not massively different, is palpable.
On a PHPBench test, the latest version is not much different from the previous one but way more performant than PHP 5, PHP 8.0-dev is already more than 7% higher than PHP 7.4.
With micro-benchmarks, PHP 7.4 has enhanced its performance even compared to last year version.
PHP 8 is yet better that it and still, it will not be released until 2021
On his blog, Andrei Avram, Back End Developer at Zenitech wrote a small stress test over looping into an array,
The test shows that the memory usage on the different version has not changed but the performance in second has increased by a lot:
Here are is results:
5.6
Execution time: 4.573347 seconds
Memory usage: 1379.250000 MB
7.0
Execution time: 1.464059 seconds
Memory usage: 360.000000 MB
7.1
Execution time: 1.315205 seconds
Memory usage: 360.000000 MB
7.2
Execution time: 0.653521 seconds
Memory usage: 360.000000 MB
7.3
Execution time: 0.614016 seconds
Memory usage: 360.000000 MB
7.4-rc
Execution time: 0.528052 seconds
Memory usage: 360.000000 MB
Installing PHP 7.4 on Docker
You can already use PHP 7.4 by installing it on your system, or you can test it by using its image on Docker.
To do that install Docker on your computer, create a folder and add the following line to a file called Dockerfile;
sudo apt-get update sudo apt-get install docker-ce docker-ce-cli containerd.io mkdir foldername cd foldername touch Dockerfile
inside the Dockerfile you would write
FROM devilbox/php-fpm-7.4:latest
Then build the container using PHP 7.4 and run it
docker build -t my-new-php-app Docker run -p -p 8080:80 my-new-php-app
Enjoy it on your browser at http://127.0.0.1:8080
Get ready for PHP 8
The new-arrived version of PHP came with a clear goal in mind, it needed to clear the code, remove as much unutilized feature as possible to have a good foundation in which we can build PHP 8 on.
And, in fact, it is PHP 8 that, even though it looks still far away, can have a great impact on the reputation of this programming language.
Several new features have already been implemented, while some others have been accepted or are still in the draft phase.
Here there is a brief description.
DOM Living Standard API
The aim here is to adapt the current DOM standard changes to the PHP language, to do so new interfaces and properties that simplify traversal and manipulation of DOM elements have been introduced.
Generate a fatal error for incompatible method signatures
This proposal plan to throw a fatal error on incompatible method signatures.
Arrays starting with a negative index
It proposes to make the code consistent by always using the index (n+1) regardless of the sign of n.
Consistent type errors for internal functions
If you pass an illegal parameter type, the PHP parser throws a TypeError.
This RFC proposed to generate TypeError for all invalid parameter types
Note that it also includes TypeError’s child ArgumentCountError in case few arguments were passed
JIT
Maybe this is the most interesting feature among all, JIT (Just in Time Compiler) promises to increase the performance of the language by converting the code into machine language and secure a deeper connection between the PHP code and the hardware of the server
There will be a lot to write about regarding PHP 8 In the next years, until then,
You can have a read at the first episode of this series if you haven’t seen it already or start implementing all these new features while using the best practices possible.
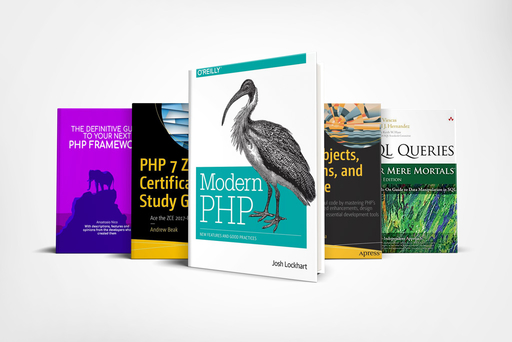