RESTful APIs with Symfony: Building Web Services
Coding (Symfony)
Discover the power of Symfony in developing RESTful APIs for seamless communication between your applications

Imagine this, you are having a great time at dinner with your friends.
All of a sudden, everyone gets the dishes they have ordered.
Everyone but you.
Minutes go by and you're still waiting for your food while every one of your friends finished their meal already.
Eventually, once everyone is done the waiter delivers a cold plate to you.
How will that experience be?
As a developer, you can provide the same horrifying experience to the visitor of your website.
In fact, in the world of web development, RESTful APIs have gained immense popularity and significance.
They serve as specialized intermediaries, just like waiters in a restaurant.
Waiters take orders and relay them to the kitchen, ensuring, (most of the time) a smooth dining experience.
Similarly, APIs receive requests from client applications and communicate with servers, facilitating efficient data exchange.
In this blog post, we'll explore building web services with Symfony, leveraging the power of APIs to create scalable and reliable applications.
So you will never leave your visitors hungry.
Understanding RESTful APIs
RESTful APIs (Representational State Transfer) are a widely adopted architectural style for designing web services.
They are based on a set of principles that promote scalability, simplicity, and interoperability.
At its core, RESTful architecture treats resources as the focal point and allows clients to perform various operations on these resources through standard HTTP methods.
The key principles of RESTful APIs include:
Uniform Interface
REST APIs provide a consistent and uniform interface for interacting with resources.
This is achieved through standardization of resource identification, representation formats (such as JSON or XML), and the use of well-defined HTTP methods.
Statelessness
RESTful APIs are stateless, meaning each request from the client contains all the necessary information needed to process it.
The server does not store any session-related data, making the system more scalable and easier to maintain.
Client-Server Separation
REST emphasizes the separation of concerns between the client and server.
The client is responsible for the user interface and presentation logic, while the server focuses on data storage, processing, and application logic.
Cacheability
RESTful APIs leverage the HTTP caching mechanisms to improve performance and reduce server load.
Responses can be marked as cacheable or non-cacheable, allowing clients or intermediary servers to store and reuse the response data when appropriate.
Using RESTful architecture for web services offers several advantages.
Firstly, it promotes scalability by allowing resources to be accessed and manipulated independently.
This modular approach enables easy addition or removal of resources without impacting the overall system.
Additionally, RESTful APIs support interoperability since they are based on well-known and widely adopted standards like HTTP.
The HTTP methods play a crucial role in RESTful API design.
They define the actions that clients can perform on resources.
The most commonly used methods are:
GET is used to retrieve a representation of a resource from the server.
POST is used to create a new resource on the server.
PUT is used to update an existing resource or create a new resource at a specific URI.
DELETE is used to remove a resource from the server.
By adhering to the appropriate use of HTTP methods, RESTful APIs ensure that the actions performed by clients are aligned with the intended behavior and semantics of the underlying resources.
In the next sections, we'll delve deeper into building web services with Symfony, leveraging the power of RESTful APIs to create efficient and scalable applications.
Getting Started with Symfony for API Development
To get started with Symfony for API development, let's explore the essential components and concepts that play a crucial role in building robust and efficient APIs.
Symfony is a powerful PHP framework known for its flexibility and extensive set of components.
It provides a solid foundation for creating RESTful APIs.
One of the key components in Symfony is the Routing component.
It allows you to define the endpoints for your API and map them to specific controllers or actions.
Here's an example of how you can define a route in Symfony:
use Symfony\Component\Routing\Annotation\Route; /** * @Route("/api/users/{id}", methods={"GET"}) */ public function getUser($id) { // Fetch user data from the database // Return the user as a JSON response }
In the above code snippet, we define a route for retrieving a user by their ID.
The `@Route` annotation specifies the URL pattern (`/api/users/{id}`) and the allowed HTTP method (`GET`).
Inside the corresponding controller action, you can implement the logic to fetch the user data from the database and return it as a JSON response.
Another crucial component in Symfony for API development is the Serializer component.
Here is a list of other Must-Have Symfony Bundles
It simplifies the process of serializing and deserializing data between PHP objects and different formats like JSON or XML.
Here's an example of how you can use the Symfony Serializer component:
use Symfony\Component\Serializer\SerializerInterface; public function createUser(Request $request, SerializerInterface $serializer) { $userData = $request->getContent(); $user = $serializer->deserialize($userData, User::class, 'json'); // Persist the user in the database // Return a success response }
In the above code snippet, we deserialize the request data (in JSON format) into a `User` object using the Symfony Serializer component.
This allows you to easily convert the incoming request payload into a structured PHP object that can be further processed and persisted in the database.
By leveraging the Symfony Routing and Serializer components, you can efficiently define API endpoints and handle data serialization/deserialization in your Symfony applications.
Designing RESTful API Endpoints
Designing RESTful API endpoints is a crucial aspect of building well-structured and intuitive APIs.
The way you model and define your resources has a significant impact on the usability, scalability, and maintainability of your API.
Proper resource modeling ensures that your API aligns with the principles of REST and provides a consistent and logical structure for accessing and manipulating data.
Each endpoint should represent a specific resource or a collection of resources in your system.
This helps in organizing and categorizing the functionality of your API in a meaningful way.
When defining the URI structure for your API endpoints, it's important to follow best practices.
A clean and hierarchical structure not only improves the readability of your API but also makes it easier for developers to navigate and understand its functionality.
Consider using nouns to represent resources and utilize the HTTP methods to specify the actions performed on those resources.
For example,
in a blog API implemented with Symfony, you could define the following endpoints using the Symfony Routing component:
use Symfony\Component\Routing\RouteCollection; use Symfony\Component\Routing\Route; $routes = new RouteCollection(); // Retrieve a specific blog post $routes->add('get_blog_post', new Route('/api/posts/{id}', [ '_controller' => 'App\Controller\PostController::get', ])); // Create a new blog post $routes->add('create_blog_post', new Route('/api/posts', [ '_controller' => 'App\Controller\PostController::create', ], [], [], '', [], ['POST'])); // Update an existing blog post $routes->add('update_blog_post', new Route('/api/posts/{id}', [ '_controller' => 'App\Controller\PostController::update', ], [], [], '', [], ['PUT'])); // Delete a blog post $routes->add('delete_blog_post', new Route('/api/posts/{id}', [ '_controller' => 'App\Controller\PostController::delete', ], [], [], '', [], ['DELETE'])); return $routes;
Versioning is another important aspect to consider in API design.
As your API evolves, it's essential to provide backward compatibility and manage changes effectively.
One approach to versioning is to include the version number in the URI, such as `/api/v1/posts`.
This allows you to introduce breaking changes in a controlled manner without affecting existing clients.
Content negotiation is a technique that allows clients to request specific representations of resources.
It involves the use of request headers, such as `Accept` and `Content-Type`, to indicate the desired data format (e.g., JSON, XML).
By supporting content negotiation, your API can cater to a wide range of clients with varying requirements.
In summary, designing RESTful API endpoints with proper resource modeling, clear URI structure, versioning, and content negotiation are vital for creating a well-structured and developer-friendly API.
Adhering to best practices in API design ensures consistency, scalability, and ease of use, empowering both API providers and consumers to interact with your system efficiently.
Handling Requests and Responses
Handling requests and responses effectively is a fundamental part of building robust and reliable APIs in Symfony.
Symfony provides powerful tools and components to handle the complexities of request handling and response generation.
When it comes to handling incoming requests, Symfony follows a controller-based approach.
Controllers are responsible for processing requests and producing responses.
To handle a request, you define a controller action that corresponds to a specific endpoint.
Within the action, you can access request data, perform necessary operations, and prepare a response.
For example, let's consider a scenario where we want to handle a POST request to create a new user in our API.
We can define a controller action using Symfony's annotations:
use Symfony\Component\HttpFoundation\Request; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\Routing\Annotation\Route; class UserController extends AbstractController { /** * @Route("/api/users", methods={"POST"}) */ public function createUser(Request $request): Response { // Access request data $data = $request->request->all(); // Perform validation and data sanitization using Symfony forms $form = $this->createForm(UserFormType::class); $form->submit($data); if (!$form->isValid()) { // Handle validation errors return $this->json(['errors' => $form->getErrors()], 400); } // Process the validated data and create a new user // ... // Return a success response return $this->json(['message' => 'User created successfully'], 201); } }
In the example above, we access the request data using the `$request` object.
Symfony's `Request` class provides various methods to retrieve different types of data, such as query parameters, request body, headers, etc.
To validate and sanitize the request data, Symfony's form component is extremely useful.
We define a form type, `UserFormType` in this case, and use it to create a form instance.
By calling `submit()` on the form with the request data, Symfony automatically validates and binds the data to the form fields.
If the form is not valid, we can handle the validation errors appropriately.
Formatting and returning responses in Symfony is straightforward.
Symfony provides multiple response types, including JSON, XML, HTML, and more.
In the example above, we use the `json()` method provided by Symfony's `AbstractController` to format the response as JSON.
We pass in the data and the HTTP status code to generate the appropriate response.
Symfony also supports content negotiation, allowing clients to specify the desired response format using the `Accept` header.
By default, Symfony's `json()` method sets the appropriate `Content-Type` header based on the requested format.
Overall, Symfony provides a comprehensive set of tools and components to handle requests and responses efficiently.
Leveraging Symfony's powerful form component, accessing request data, and generating responses with ease allows you to focus on implementing your API's business logic effectively.
Authentication and Authorization in RESTful APIs
Authentication and authorization are crucial aspects of API security.
They ensure that only authenticated and authorized users have access to sensitive resources and operations.
Symfony provides robust mechanisms to handle authentication and authorization, making it easier to secure your API endpoints effectively.
Symfony supports various authentication methods, including JSON Web Tokens (JWT) and OAuth2.
These authentication mechanisms allow you to validate the identity of the API client and grant access based on the provided credentials.
JSON Web Tokens (JWT) is a popular authentication method in the API world.
It allows clients to authenticate by exchanging digitally signed tokens.
Symfony provides the LexikJWTAuthenticationBundle, which integrates JWT authentication seamlessly.
With this bundle, you can generate and validate JWT tokens, define token expiration and refresh strategies, and configure token encryption settings.
OAuth2 is another widely used authentication protocol for API security.
Symfony provides the FOSOAuthServerBundle, which allows you to set up an OAuth2 server and implement authentication and authorization flows, such as authorization code, implicit, and client credentials grants.
OAuth2 provides a flexible and secure way to authenticate users and grant access to protected resources.
Once authentication is in place, it's essential to control access to specific resources and operations based on user roles and permissions.
Symfony offers a robust access control system that allows you to define fine-grained access rules using security annotations or configuration files.
Symfony's access control system is based on voters and roles.
Voters are responsible for making access decisions based on rules defined in your application.
Roles represent different levels of authorization that can be assigned to users.
By configuring access control rules, you can define which roles have access to specific routes or resources.
For example, let's say we have an API endpoint that allows users to delete a post.
We can restrict access to this endpoint only to users with the "ROLE_ADMIN" role:
/** * @Route("/api/posts/{id}", methods={"DELETE"}) * @IsGranted("ROLE_ADMIN") */ public function deletePost(Post $post): Response { // Delete the post // ... }
In the example above,
the `@IsGranted` annotation ensures that only users with the "ROLE_ADMIN" role can access the `deletePost` action.
Symfony's access control system automatically checks the user's roles and denies access if the user doesn't meet the required role.
By leveraging Symfony's authentication mechanisms and access control system, you can enforce strong security measures in your API.
Implementing authentication ensures that only valid users can access your API, while authorization controls what each user can do within the API.
These measures contribute to maintaining the confidentiality and integrity of your resources, protecting them from unauthorized access and misuse.
Conclusion
In conclusion, building RESTful APIs with Symfony allows for robust and scalable web services.
As waiters in a restaurant even for us developers following best practices is very important.
These practices go from endpoint design, to request handling, and security to ensure exceptional user experiences.
Stay tuned for more Symfony development insights by subscribing to our newsletter and exploring our website for valuable resources and tutorials.
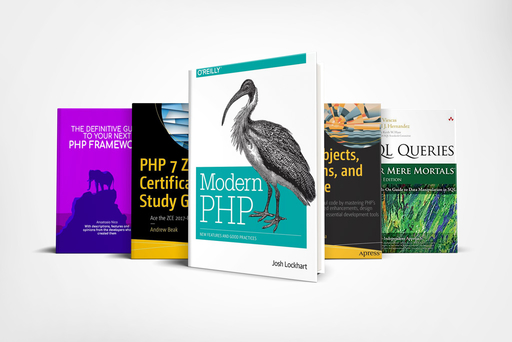