Error Handling in Symfony: 3 Practices you must know
Coding (Symfony)
Become a Symfony error-handling expert with this practical guide. Boost your coding skills and deliver seamless web apps
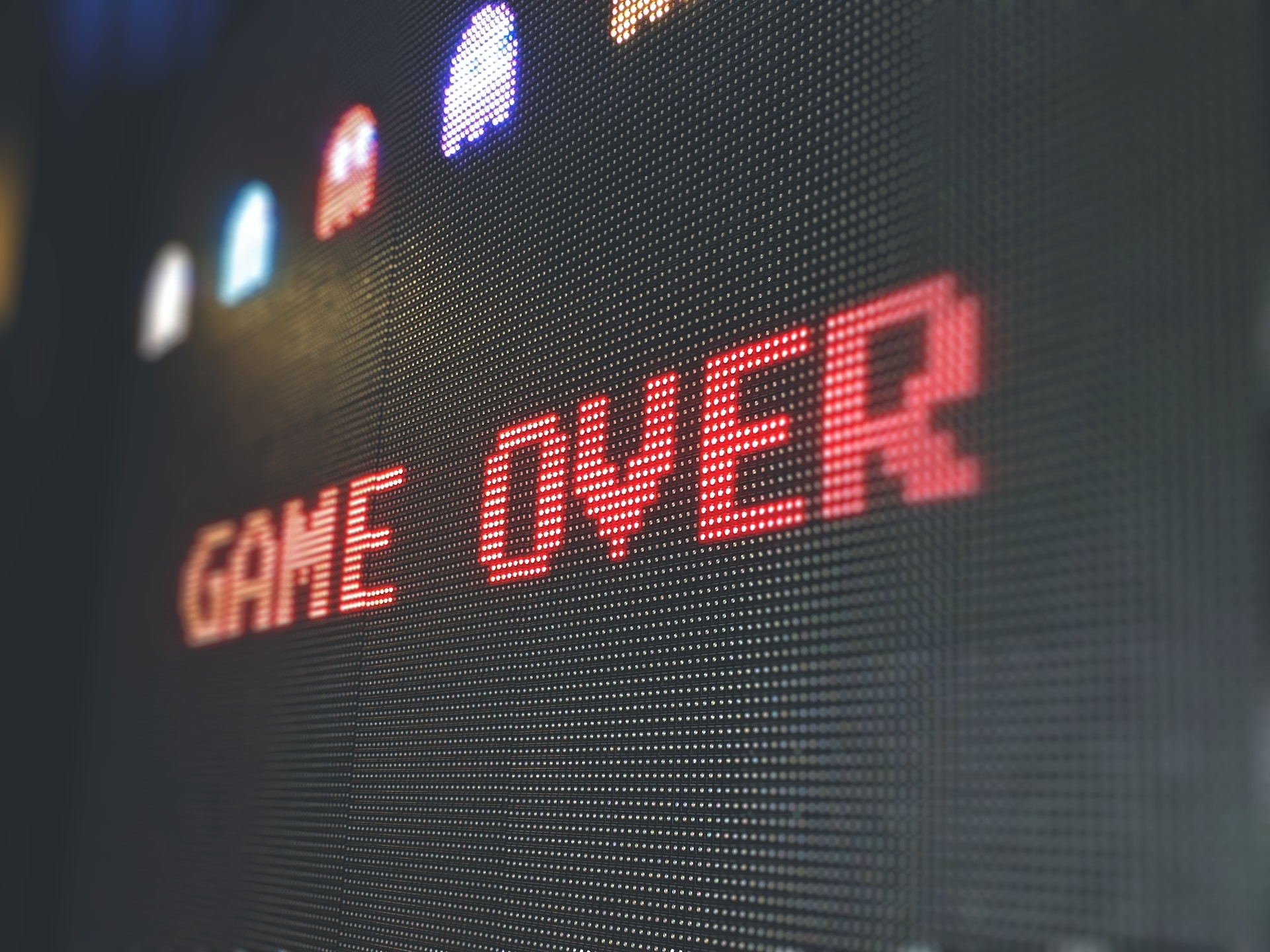
Have you ever found yourself in a sticky situation?
As a web developer, it is a common thing and this is where today's story starts.
Joseph is a passionate web developer.
He is working at a company that sells fitness supplements, Joseph was coding a new feature for the company's website when he accidentally made a critical error.
The result of this mistake was that instead of sending 2kg of protein powder to a client, the system sent a whopping 200kg!
The unintended delivery left Joseph red-faced and the company with a lot of explaining to do.
But from this unfortunate mishap, Joseph learned an invaluable lesson about the importance of error handling.
He realized that even a small oversight could lead to significant consequences.
Since then, Joseph has made it his mission to always check for errors and employ the right techniques to ensure smooth and reliable software delivery.
And that is why in this blog post, we will delve into Symfony and explore the best practices and techniques for error handling.
By learning from Joseph's experience and exploring Symfony's powerful error-handling capabilities, you'll gain the knowledge and skills to elevate your web development journey.
Let's dive into error handling in Symfony!
Understanding Error Types and Levels in Symfony 6
When working with Symfony, it's essential to understand the various types of errors that can occur during the development process.
These errors are classified into three main categories: Notices, Warnings, and Errors.
Notices
Notices are the mildest form of error in Symfony.
They are often non-critical issues that indicate potential problems in your code but do not necessarily affect the overall functionality of your application.
Warnings
Warnings, as the name suggests, indicate more serious issues than Notices.
These errors might have a slight impact on your application's functionality, but they don't necessarily break it.
Errors
Errors are the most severe type of issue in Symfony.
They indicate critical problems that can lead to the failure of your application.
When an error occurs, your application might stop working altogether.
To effectively handle these errors, Symfony provides different levels of error reporting that you can configure based on your development environment.
These reporting levels include:
Development Environment
In the development environment, Symfony is set to display all types of errors, including Notices, Warnings, and Errors, to help developers identify and fix issues quickly.
Production Environment
In the production environment, Symfony is configured to display only Errors to avoid exposing sensitive information to users and provide a more user-friendly experience.
Symfony's error reporting levels can be adjusted in the configuration files to suit your specific needs.
By understanding the different error types and levels, you'll be better equipped to identify and address potential issues in your Symfony applications, ensuring a smooth and reliable user experience.
Utilizing Symfony's Exception Component
Symfony's Exception Component is a powerful tool that allows developers to handle errors and exceptional situations with ease.
Let's explore how you can make the most of this component in your Symfony applications.
The Exception Component in Symfony provides a standardized way to deal with errors and exceptions.
It allows you to create custom exceptions tailored to specific use cases, making your error handling more organized and expressive.
In Symfony, you can create your own custom exceptions by extending the base `Exception` class.
This enables you to define exceptions that are meaningful and relevant to your application's domain.
Let's take an example where you're building an e-commerce application, and you want to handle specific exceptions for product-related errors:
use Symfony\Component\Config\Exception\ExceptionInterface; class ProductNotFoundException extends \Exception { public function __construct(string $message = "Product not found.", int $code = 0, \Throwable $previous = null) { parent::__construct($message, $code, $previous); } }
To gracefully handle exceptions in Symfony, you can use try-catch blocks.
The `try` block contains the code that might throw an exception, and the `catch` block allows you to handle the exception appropriately:
try { // Code that may throw an exception // For example, querying a product from the database $product = $productRepository->find(123); if (!$product) { throw new ProductNotFoundException("Product with ID 123 not found."); } } catch (ProductNotFoundException $e) { // Handle the exception // For example, log the error and show a user-friendly message $logger->error("Product not found: " . $e->getMessage()); $response = new Response("Product not found. Please try again later.", 404); }
In Symfony, you can create an ExceptionHandler to centralize the handling of exceptions.
This way, you can have consistent error responses throughout your application:
use Symfony\Component\HttpKernel\Exception\HttpExceptionInterface; use Symfony\Component\HttpFoundation\Response; use Symfony\Component\HttpKernel\Event\ExceptionEvent; class ExceptionHandler { public function onKernelException(ExceptionEvent $event) { $exception = $event->getThrowable(); if ($exception instanceof HttpExceptionInterface) { $statusCode = $exception->getStatusCode(); $message = $exception->getMessage(); } else { $statusCode = Response::HTTP_INTERNAL_SERVER_ERROR; $message = "An unexpected error occurred."; } // Log the error and respond with a user-friendly message $logger->error("Exception: " . $message); $response = new Response($message, $statusCode); $event->setResponse($response); } }
By utilizing Symfony's Exception Component and implementing proper error handling techniques, you can ensure that your Symfony application gracefully handles errors and exceptions, providing a more reliable and user-friendly experience for your users.
PHP 8 Enhancements for Error Handling in Symfony 6
Let's expand our view of a second and focus on PHP 8
This version of the language has brought several advancements to the language, including improvements in error handling.
Let's take a look at these features and how they can benefit your Symfony application, especially if you're working on an e-commerce like a supplements-selling company!
PHP 8 introduces a new feature known as 'Throw Expressions.'
This allows 'throw' to be used in places where only expressions are allowed.
This means you can now use 'throw' in more contexts than before, making your error-handling code more concise and readable.
Match expressions
Match expressions are a new feature in PHP 8 that can simplify your error-handling logic.
Instead of using a switch statement or a series of if-else statements, you can use a match expression to check for different error conditions.
Here's how:
$orderStatus = $order->getStatus(); $message = match($orderStatus) { 'processed' => 'Your order has been processed.', 'shipped' => 'Your order has been shipped.', 'delivered' => 'Your order has been delivered.', default => 'Unknown order status.', }; if ($message === 'Unknown order status.') { throw new Exception($message); } echo $message;
PHP 8's throw expressions allow you to throw exceptions within arrow functions, ternary operations, and coalesce operations.
This can make your error-handling code more concise and intuitive:
$product = $productRepository->find($productId) ?? throw new ProductNotFoundException('Product not found.'); // Proceed with processing the order
In this example, if the `find` method returns null (meaning the product was not found), a `ProductNotFoundException` is thrown.
Otherwise, the found product is assigned to the `$product` variable.
These new error-handling features in PHP 8 can help you write more readable, and expressive error-handling code in your Symfony applications.
The next time you're working on a PHP project consider leveraging these features for better error management.
Effective Debugging Techniques in Symfony 6
Debugging is a crucial skill for any web developer, and Symfony provides powerful tools to make the process efficient and effective.
Let's explore some effective debugging techniques to help you identify and resolve common errors in your Symfony application.
When working on a Symfony project, you may encounter common errors like syntax errors, undefined variables, or issues with database queries.
To identify these errors, enable Symfony's debug mode in your development environment.
This mode provides detailed error messages with stack traces, making it easier to pinpoint the exact location of the issue.
// Enable debug mode in development environment // app/config/packages/dev/framework.yaml framework: # ... debug: true
With debug mode enabled, if there's an error in your code, Symfony will display a detailed error page with information about the error's source and the execution path leading up to it.
Symfony comes with powerful built-in debugging tools, such as the Profiler and the Debug Toolbar, which can significantly speed up the debugging process.
The Profiler provides detailed information about the performance of your application and the database queries executed during a request.
You can access it by visiting the `/_profiler` route in your browser.
The Debug Toolbar appears at the bottom of the page and displays helpful information about the request, including executed queries, HTTP status, and route parameters.
// Accessing the Profiler and Debug Toolbar in a Twig template {{ render(url('_profiler', {'token': app.request.attributes.get('_profiler_token')})) }}
Effective debugging involves adopting some best practices to streamline the process:
Debugging Step-by-Step
Avoid trying to fix multiple issues simultaneously.
Focus on one problem at a time and debug it step-by-step to identify the root cause.
Log Messages
Use Symfony's logging system to record relevant information during development.
You can log messages to investigate the application's behavior and track potential issues.
// Example of logging in Symfony $this->logger->info('Order processing started.', ['order_id' => $order->getId()]);
Dumping Variables
Use Symfony's `dump()` function to inspect the contents of variables and objects.
This can help you better understand their values and structures during runtime.
// Example of dumping variables in Symfony dump($order);
By employing these debugging techniques and leveraging Symfony's debugging tools, you can efficiently identify and troubleshoot errors in your Symfony 6 application, ensuring smooth operations in any Symfony project.
Handling Errors in Specific Use Cases
Error handling in Symfony 6 goes beyond traditional web applications and extends to various use cases, including REST APIs, form handling, and background processes.
Let's explore how to handle errors effectively in these specific scenarios, ensuring a smooth experience for the users.
When building RESTful APIs with Symfony, it's essential to provide meaningful error responses to clients.
One common approach is to use Symfony's exception handling to return appropriate error codes and messages.
For example, in the context of an order API, we can create a custom exception for invalid orders:
// Custom exception for invalid orders in a API class InvalidOrderException extends \RuntimeException {} // In the controller action for handling orders try { // Process the order $order = $orderService->processOrder($requestData); } catch (InvalidOrderException $e) { // Return a JSON response with error details return $this->json(['error' => 'Invalid order data.'], 400); }
By utilizing custom exceptions and returning appropriate JSON responses, your API clients will receive clear error messages when they encounter issues while interacting with the API.
Symfony provides robust form handling and validation capabilities to ensure data integrity in your application.
When handling form submissions, you can check for validation failures and display error messages to users.
// In the controller action for processing the form $form = $this->createForm(OrderFormType::class, $order); $form->handleRequest($request); if ($form->isSubmitted() && $form->isValid()) { // Process the order $orderService->processOrder($order); // Redirect to a success page return $this->redirectToRoute('order_success'); } // Render the form template with error messages return $this->render('order_form.html.twig', [ 'form' => $form->createView(), ]);
Symfony's form validation will automatically handle validation failures and display error messages for invalid fields, guiding users to correct their input.
In some scenarios, error handling for asynchronous and background processes may differ from traditional request-response flows.
When dealing with tasks like processing orders or generating reports in the background, you need to handle errors in a way that doesn't disrupt the main application flow.
// Example of error handling in an asynchronous process try { // Process bulk orders $orderService->processBulkOrders($bulkData); } catch (\Throwable $e) { // Log the error for further investigation $this->logger->error('Bulk order processing failed.', ['error' => $e->getMessage()]); // Optionally, take appropriate recovery actions }
In such cases, it's crucial to log errors for later review, and, depending on the specific use case, you might implement retries or queuing mechanisms to ensure robustness in handling errors.
By understanding and implementing error-handling techniques in these specific use cases, you can create more resilient and user-friendly applications.
Conclusion
Mastering error handling in Symfony 6 is of utmost importance for web developers like our friend Joseph, who learned from his past mistake and now ensures that his protein powder orders are processed flawlessly.
Understanding different error types, utilizing Symfony's exception component, and taking advantage of PHP 8's enhancements are crucial steps toward robust and efficient error handling.
Remember, error handling goes beyond merely fixing bugs; it's about creating a smooth and reliable experience for your application users.
By implementing the best practices and techniques we've explored, you can confidently handle errors and provide helpful feedback to users, enhancing their overall interaction with your Symfony applications.
As you continue your web development journey, I encourage you to embrace continuous learning and improvement in error-handling skills.
The world of Symfony and PHP is constantly evolving, and staying up-to-date with the latest practices will make you a more effective and competent developer.
If you want to learn more about Symfony and stay informed about the latest articles, be sure to subscribe to my newsletter.
I regularly share insights and tips on Symfony and other web development topics, aiming to help you become an even better developer.
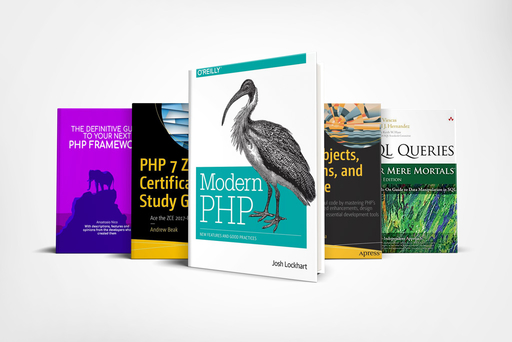